KotlinのIntentによりAndroidアプリで画面遷移する方法を現役エンジニアが解説【初心者向け】
初心者向けにKotlinのIntentによりAndroidアプリで画面遷移する方法について現役エンジニアが解説しています。IntentとはAndroidアプリで他の機能や画面との橋渡しを行うっ仕組みです。画面遷移や別の機能を起動することができます。明示的に指定する方法・Android OSに任せる方法を解説します。
テックアカデミーマガジンは受講者数No.1のプログラミングスクール「テックアカデミー」が運営。初心者向けにプロが解説した記事を公開中。現役エンジニアの方はこちらをご覧ください。 ※ アンケートモニター提供元:GMOリサーチ株式会社 調査期間:2021年8月12日~8月16日 調査対象:2020年8月以降にプログラミングスクールを受講した18~80歳の男女1,000名 調査手法:インターネット調査
KotlinのIntentによりAndroidアプリで画面遷移する方法について、TechAcademyのメンター(現役エンジニア)が実際のコードを使用して初心者向けに解説します。
初心者向けにKotlinの入門向けサイトをまとめた記事もありますので読んでみてください。
なお本記事は、TechAcademyのオンラインブートキャンプ、Androidアプリ開発講座の内容をもとに作成しています。

今回は、Androidアプリ開発に関する内容だね!
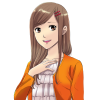
どういう内容でしょうか?

KotlinのIntentによりAndroidアプリで画面遷移する方法について詳しく説明していくね!
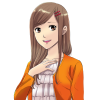
お願いします!
目次
Intentとは
Androidアプリで他の機能や画面との橋渡しを行うのがIntentです。
Intentはアプリ内で別の画面に遷移したり、別の機能を起動したりといった、何らかの「目的」を実現するための仕組みとして利用されています。
Intentという単語には「目的」や「意図」といった意味があります。 Intentには「明示的Intent」と「暗黙的Intent」の2種類があります。
- 明示的Intent:対象となる画面や機能を明示的に指定する方法です。今回の記事では明示的Intentについて説明します
- 暗黙的Intent:「写真を撮りたい」、「メールを送りたい」といった「意図」だけを指定する方法です。どの機能が起動するかはAndroid OSに任せます
Javaのコードとの差異
明示的Intentでは対象の画面などをクラスオブジェクトで指定します。KotlinとJavaではクラスオブジェクトの指定方法が異なるので注意しましょう。
Java
Intent intent = new Intent(this, SubActivity.class);
Kotlin
val intent = Intent(this, SubActivity::class.java)
画面遷移処理を書いてみよう
今回のサンプルプログラムではIntentの使い方を確認します。合わせて画面間で値を受け渡す方法についても例示しています。
事前にプロジェクトを「Empty Activity」で新規作成し、新たにEmpty Activityで「SubActivity」を追加しておきます。はじめにそれぞれの画面のレイアウトファイルを修正します。
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <EditText android:id="@+id/editText" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="100dp" android:ems="10" android:inputType="textPersonName" android:text="" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="16dp" android:text="SubActivityに移動" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/editText" /> </androidx.constraintlayout.widget.ConstraintLayout>
activity_sub.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".SubActivity"> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="100dp" android:text="aaa" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="16dp" android:text="戻る" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView" /> </androidx.constraintlayout.widget.ConstraintLayout>
次にIntentを使用した画面遷移のコードを記述していきます。
MainActivityのonCreate関数にソースコードを追記します。全体は以下のようになります。putExtraでSubActivityに受け渡す値を設定しています。
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) button.setOnClickListener { // 起動する対象をクラスオブジェクトで指定する val intent = Intent(this, SubActivity::class.java) intent.putExtra("VALUE", editText.text.toString()) startActivity(intent) } }
SubActivityでは受け取った値を画面に表示しています。またボタンが押された際にSubActivityを終了するようにしています。
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_sub) val value1 = intent.getStringExtra("VALUE") textView.text = "入力された値:${value1}" button.setOnClickListener { finish() } }
実行結果は以下のとおりです。MainActivityでtestと入力してボタンをクリックします。
SubActivityでは受け渡された値が表示されていることを確認できます。
まとめ
今回の記事ではIntentの使い方について学習しました。
監修してくれたメンター
太田和樹(おおたかずき)
ITベンチャー企業のPM兼エンジニア。 普段は主に、Web系アプリケーション開発のプロジェクトマネージャーとプログラミング講師を行っている。守備範囲はフロントエンド、モバイル、サーバサイド、データサイエンティストと幅広い。その幅広い知見を生かして、複数の領域を組み合わせた新しい提案をするのが得意。 開発実績:画像認識技術を活用した駐車場混雑状況把握(実証実験)、音声認識を活用したヘルプデスク支援システム、Pepperを遠隔操作するアプリの開発、大規模基幹系システムの開発・導入マネジメント。 地方在住。仕事のほとんどをリモートオフィスで行う。通勤で消耗する代わりに趣味のDIYや家庭菜園、家族との時間を楽しんでいる。 |
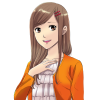
内容分かりやすくて良かったです!

ゆかりちゃんも分からないことがあったら質問してね!
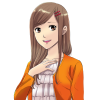
分かりました。ありがとうございます!
TechAcademyでは、初心者でも最短4週間で、Kotlinの技術を使ってAndroidアプリ開発を習得できる、オンラインブートキャンプを開催しています。
また、現役エンジニアから学べる無料体験も実施しているので、ぜひ参加してみてください。