Javaでカウントダウンタイマーを作成する方法を現役エンジニアが解説【初心者向け】
初心者向けにJavaでカウントダウンタイマーを作成する方法について解説しています。ここではjavafx.animation.Timelineクラスを使ってカウントダウンタイマーを作成します。基本の設計と処理の流れをサンプルコードで確認しましょう。
テックアカデミーマガジンは受講者数No.1のプログラミングスクール「テックアカデミー」が運営。初心者向けにプロが解説した記事を公開中。現役エンジニアの方はこちらをご覧ください。 ※ アンケートモニター提供元:GMOリサーチ株式会社 調査期間:2021年8月12日~8月16日 調査対象:2020年8月以降にプログラミングスクールを受講した18~80歳の男女1,000名 調査手法:インターネット調査
Javaでカウントダウンタイマーを作成する方法について解説します。実際にプログラムを書いて説明しているので、ぜひ理解しておきましょう。
そもそもJavaについてよく分からないという方は、Javaとは何なのか解説した記事を読むとさらに理解が深まります。
なお本記事は、TechAcademyのオンラインブートキャンプJava講座の内容をもとに作成しています。

今回は、Javaに関する内容だね!
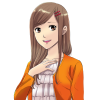
どういう内容でしょうか?

カウントダウンタイマーを作成する方法について詳しく説明していくね!
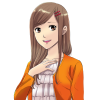
お願いします!
カウントダウンタイマーのプログラムの仕組み
javaFXを利用してカウントダウンタイマーを作成します。カウントダウンタイマーは、プルダウンで分数、秒数を選択しスタートボタンを押すとカウントダウンがはじまります。0分0秒となるまでか、リセットボタンが押下されるまで継続します。
カウントダウンタイマーに利用できるJavaのクラスなど
カウントダウンしている要素を画面に描写するにはjavafx.animation.Timelineクラスを使います。一定間隔で繰り返す処理をアニメーションとして定義することができます。今回は1秒(1000ミリ秒)間隔で画面に残り時間を表示するようにします。
実際に書いてみよう
import javafx.animation.KeyFrame; import javafx.animation.Timeline; import javafx.application.Application; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.geometry.Insets; import javafx.geometry.Orientation; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.ComboBox; import javafx.scene.control.Label; import javafx.scene.layout.FlowPane; import javafx.scene.layout.TilePane; import javafx.scene.text.Font; import javafx.stage.Stage; import javafx.util.Duration; public class Sample extends Application { // 画面上部ペイン FlowPane flowTop; // 画面下部ペイン FlowPane flowBottom; // 分数選択コンボボックス ComboBox<Integer> comboMin; // 分ラベル Label labelMin = new Label("分"); // 秒数選択コンボボックス ComboBox<Integer> comboSec; // 秒ラベル Label labelSec = new Label("秒"); // スタートボタン Button buttonStart = new Button("スタート"); // リセットボタン Button buttonReset = new Button("リセット"); // タイマー部分 分表示ラベル Label TimerMin = new Label(); // タイマー部分 分ラベル Label labelTimerMin = new Label("分"); // タイマー部分 秒表示ラベル Label TimerSec = new Label(); // タイマー部分 秒ラベル Label labelTimerSec = new Label("秒"); // タイマー部分 終了ラベル Label labelTimerFinish = new Label(""); //アニメーションクラス Timeline timer; public static void main(String[] args) { launch(); } @SuppressWarnings("static-access") public void start(Stage stage) { ObservableList<Integer> data = FXCollections.observableArrayList(); for (int i = 0; i < 60; i++) { data.add(i, i); } // 分数選択コンボボックス comboMin = new ComboBox<Integer>(data); comboMin.setOnAction((ActionEvent) -> { TimerMin.setText(String.format("%02d", comboMin.getValue())); }); comboMin.setValue(0); // 秒数選択コンボボックス comboSec = new ComboBox<Integer>(data); comboSec.setOnAction((ActionEvent) -> { TimerSec.setText(String.format("%02d", comboSec.getValue())); }); reset(); // 画面上部ペインを作成 flowTop = new FlowPane(); flowTop.setPadding(new Insets(10, 10, 10, 10)); flowTop.setVgap(1); flowTop.setHgap(1); // 画面上部の要素をペインへ配置 flowTop.getChildren().add(comboMin); flowTop.getChildren().add(labelMin); flowTop.getChildren().add(comboSec); flowTop.getChildren().add(labelSec); flowTop.getChildren().add(buttonStart); flowTop.getChildren().add(buttonReset); // 画面下部ペインを作成 flowBottom = new FlowPane(); TimerMin.setFont(new Font(50)); TimerMin.setText("00"); TimerSec.setFont(new Font(50)); TimerSec.setText("00"); // 画面下部要素を追加 flowBottom.getChildren().add(TimerMin); flowBottom.getChildren().add(labelTimerMin); flowBottom.getChildren().add(TimerSec); flowBottom.getChildren().add(labelTimerSec); flowBottom.getChildren().add(labelTimerFinish); buttonStart.setOnAction((ActionEvent) -> { buttonStart.setDisable(true); buttonReset.setDisable(false); // アニメーションの定義(1000ミリ秒間隔で描写する) timer = new Timeline(new KeyFrame(Duration.millis(1000), new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent event) { // 秒数、分数の繰り下げ if (TimerSec.getText().equals("00")) { if (TimerMin.getText().equals("00")) { // 全て0になったら終了ラベルを表示 labelTimerFinish.setText(" 終了!!"); } else { TimerSec.setText("59"); TimerMin.setText(String.format("%02d", Integer.parseInt(TimerMin.getText()) - 1)); } } else { TimerSec.setText(String.format("%02d", Integer.parseInt(TimerSec.getText()) - 1)); } } })); // アニメーションを無限に繰り返す timer.setCycleCount(Timeline.INDEFINITE); // アニメーション開始 timer.play(); }); // リセットボタンが押下された場合 buttonReset.setOnAction((ActionEvent) -> { // タイマーしょ終了する timer.stop(); // 初期化処理を行う reset(); }); // ペインに画面上部、下部のペインを追加 TilePane tile = new TilePane(Orientation.VERTICAL); tile.getChildren().add(flowTop); tile.getChildren().add(flowBottom); // 画面にスタック・ペインを追加 stage.setScene(new Scene(tile, 300, 200)); // 画面の表示 stage.show(); } void reset() { // リセットボタンを非活性化 buttonReset.setDisable(true); // スタートボタンを活性化 buttonStart.setDisable(false); // プルダウンの値を初期化 comboMin.setValue(0); comboSec.setValue(0); // タイマー部分の表示を初期化 TimerSec.setText("00"); TimerMin.setText("00"); // 終了ラベルを初期化 labelTimerFinish.setText(""); } }
監修してくれたメンター
長屋雅美
独立系SIerで7年勤務後、現在はフリーのエンジニアとして自宅をオフィスとして活動しています。 |
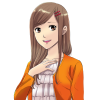
内容分かりやすくて良かったです!

ゆかりちゃんも分からないことがあったら質問してね!
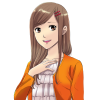
分かりました。ありがとうございます!
TechAcademyでは、初心者でもJavaやServletの技術を使ってWebアプリケーション開発を習得できるオンラインブートキャンプJava講座を開催しています。
挫折しない学習方法を知れる説明動画や、現役エンジニアとのビデオ通話とチャットサポート、学習用カリキュラムを体験できる無料体験も実施しているので、ぜひ参加してみてください。